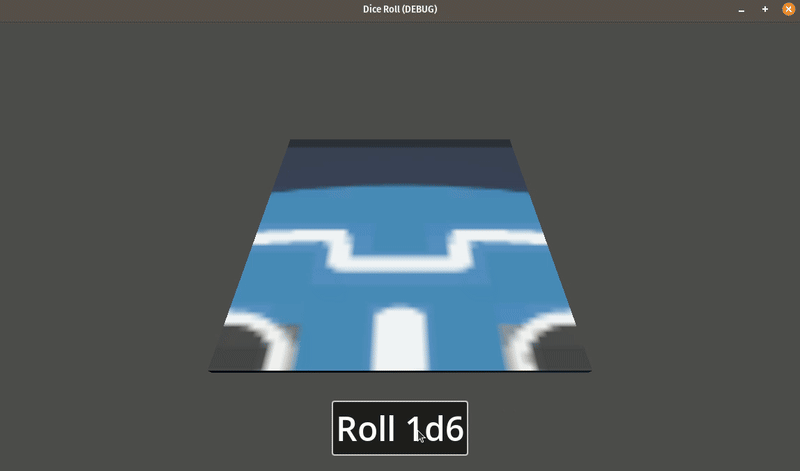
While thinking about the project for Brackeys Game Jam 2025.1, I decided to go for a shameful copy of Balatro, but instead of a deck of cards the player would roll dice to score points. The project name: Snake Eyes & The Dicey Vice (Yes, I am in a KGLW vibe lately)
I scrapped the project because, at the end of the day, it didn’t follow the theme. But one major point emerged during development: How do I even implement the logic for a dice roll? Who do I find out the result of the roll? Can I somehow make it customizable?
Note: Looking to my code while I write this post, I notice how the implementation was an absolute garbage. Use it wisely and improve it without moderation.
Project repository: https://github.com/fabrb/diceroll-godot
The project on this post will have two major scenes:
- D6
- Table
And also will have scripts for:
- Dice physics
- Updating dice face values (Optional)
- Handling UI
- Rolling the dice
- Validate score
D6 Scene
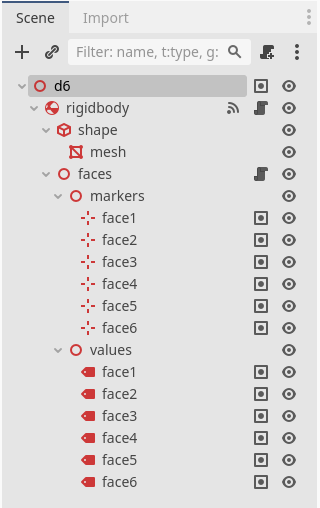
This scene has a container holding all the information for it. It’s notable to show here:
Group name
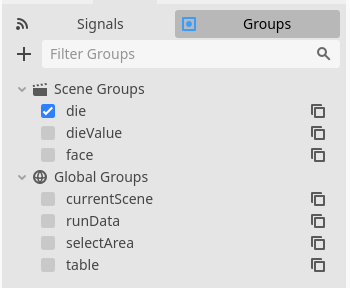
Metadata
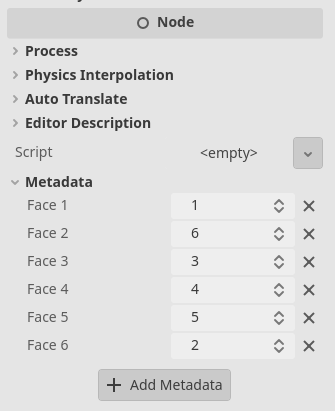
The shape node is a CollisionShape3D, and it child mesh being a MeshInstance3D. The mesh by itself just utilizes the Surface Material Override for rendering our icon.svg
placeholder.
Faces nodes is a more interesting little beast.
The original plan was to make every single face number customizable. That’s why there is a Metadata with values. Those values would be read right after the initialization, searching for the name (face1, face2…) and related value.
Each Label3D in values has the group name dieValue
.
Why all that? This is where our first script comes to help: dieValueRender.gd
extends Node3D
func _ready() -> void:
for die in get_tree().get_nodes_in_group("die"):
if (die == self.get_parent().get_parent()):
for value: Label3D in get_tree().get_nodes_in_group("dieValue"):
if (value.get_parent().get_parent() == self):
value.text = str(die.get_meta(value.name))
Basically: For each die active in the die group, check for it parent. Later, for each face in dieValue
group, I check if the parent is the current node running the script. If everything align, I can finally get the face value in the d6 node meta.
All that optional logic can be streamlined, but it allows me customize the D6 with random values:
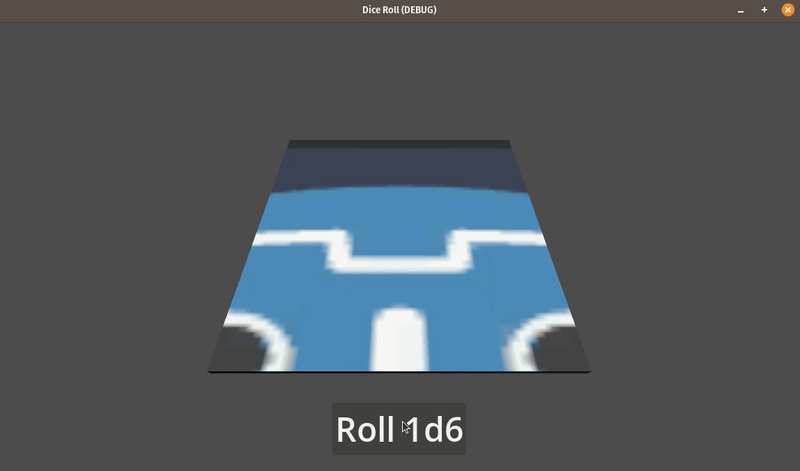
Next up: Logic for the die, found in dieMovement.gd
Initially, we have these variables:
var isActive: bool = true
var isMoving: bool = false
var isAboveMinVelocity: bool = false
var isOnGround: bool = false
The _ready()
function will store some useful data for each face and it value. I am doing it so for a future check when the roll is resolved.
func _ready() -> void:
for face in get_tree().get_nodes_in_group("face"):
if (face.get_parent().get_parent().get_parent() == self):
faces.append(face)
for value in get_tree().get_nodes_in_group("dieValue"):
if (value.get_parent().get_parent().get_parent() == self):
values.append(value)
The rollDie()
function is pretty straight forward. It applies some random forces, rolling the dice.
func rollDie() -> void:
var rng: RandomNumberGenerator = RandomNumberGenerator.new()
self.apply_impulse(Vector3.UP * rng.randf_range(10, 11))
self.apply_impulse(Vector3.LEFT * rng.randf_range(-1.6, 1.6))
self.apply_impulse(Vector3.BACK * rng.randf_range(-1.6, 1.6))
self.apply_torque(Vector3(rng.randi_range(-100, 100), rng.randi_range(-100, 100), rng.randi_range(-100, 100)))
isMoving = true
To validate if the dice is on ground or not, I check if the body entering or exiting the contact with it has the “table” group:
func _on_body_entered(body: Node) -> void:
for group in body.get_groups():
if (group.get_basename() == "table"):
isOnGround = true
return
func _on_body_exited(body: Node) -> void:
for group in body.get_groups():
if (group.get_basename() == "table"):
isOnGround = false
return
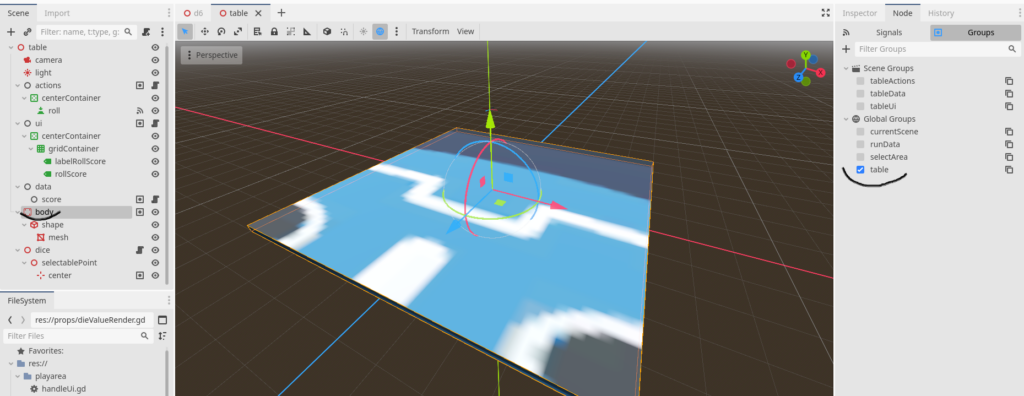
Note: It’s valid to say, you need to check the Contact Monitor option on your node, so it can observe how is touching it.
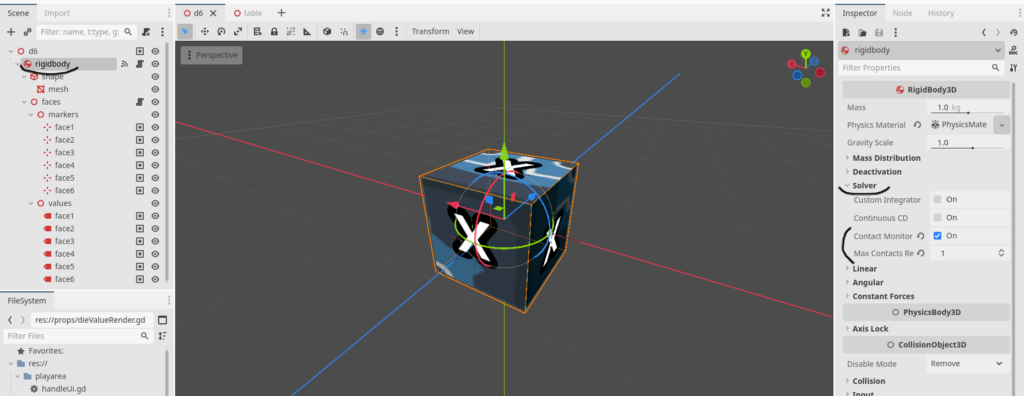
Finally, we are going to check our _physics_process()
and findFaceSelected()
functions:
func _physics_process(delta: float) -> void:
if not isActive:
return
isAboveMinVelocity = linear_velocity.length() >= 0.001
if (isMoving and not isAboveMinVelocity and isOnGround):
isActive = false
isMoving = false
for nodesData in get_tree().get_nodes_in_group("tableData"):
nodesData.call("setRollValue", int(findFaceSelected().text))
for nodesData in get_tree().get_nodes_in_group("tableActions"):
nodesData.call("finishRoll")
First things first. After checking if the D6 is inactive, we take a look for it velocity. I used the magic value of 0.001 here, but you can make it variable for better control.
After that, if our D6 is not above the minimal velocity, is on ground and has the current state of isMoving, we begin out logic to find out the rolled value and tell other nodes about the result.
The D6 tells the node with “tableData” group: “Hey, set the current result with this value“, and the node the “tableActions” group: “It is done“.
But to determine the result value, we need to take a look at our findSelectedFace()
function:
var faces: Array[Marker3D] = []
var values: Array[Label3D] = []
func findFaceSelected() -> Label3D:
var bestValue: float = -1
var bestFace: Node = null
for face in faces:
var pos: float = face.global_transform.origin.dot(Vector3.UP)
if (pos > bestValue):
bestValue = pos
bestFace = face
for value in values:
if (value.name == bestFace.name):
return value
return null
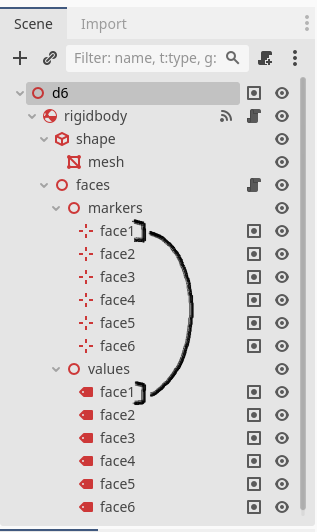
For each face in our die, we compare the marker position with a vector pointing upwards. The best face will have the greatest value.
After that, we find the label based on the name of the best face, and return it to the original caller (_physics_process
())
For example, if markers.face1 was the best face here, we search a node with the same name under values, being values.face1 in this case. This node has the text property equal to “3”. So at the end of our day we now the result value will be 3.
Is it convoluted? Yes.
Does it work: Also yes.
Table scene
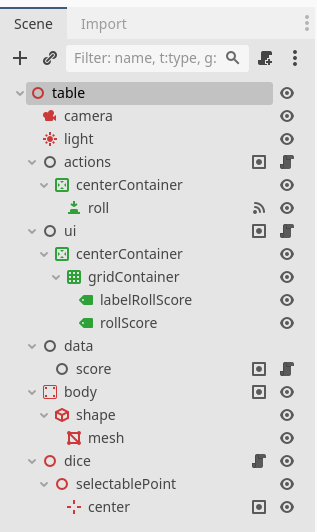
Starting simple, lets take a look at handleUi.gd
under actions:
extends Node
@onready var rollBtn: Button = $centerContainer/roll
func startRoll() -> void:
rollBtn.disabled = true
func finishRoll() -> void:
rollBtn.disabled = false
We only tell the editor to disable the button if the roll has started, or to enable it when it concludes. Pretty simple.
And our button will send a signal to another node when it was pressed. We are going to get there in a minute.
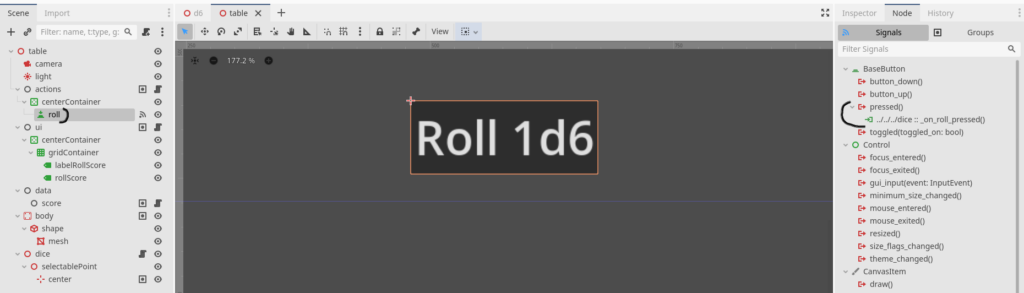
Next up, the tableUi.gd
script under ui:
extends Node
@onready var rollScore: Label = $centerContainer/gridContainer/rollScore
@onready var container: CenterContainer = $centerContainer
func _ready() -> void:
rollScore.text = "0"
container.visible = false
func updateRollScore(rollValue: int) -> void:
rollScore.text = str(rollValue)
func hideScore() -> void:
container.visible = false
func showScore() -> void:
container.visible = true
We just show or hide useful information based on the roll state. Also pretty straight forward.
Now up: validateScore.gd
under data:
extends Node
var rollScore: int = 0
func rollStarted(numberOfDice: int) -> void:
for nodesData in get_tree().get_nodes_in_group("tableUi"):
nodesData.call("hideScore")
func setRollValue(value: int) -> void:
for nodesData in get_tree().get_nodes_in_group("tableUi"):
nodesData.call("updateRollScore", value)
nodesData.call("showScore")
The rollStarted() function is called when we are starting our roll, so we can hide the UI, and setRollValue() when the D6 finishes moving. Not complicated.
Last but not least, our playDice.gd
script under dice:
extends Node3D
var dieScene: Node
var diceScenes: Array[Node]
@onready var centerPoint: Marker3D = $selectablePoint/center
func _on_roll_pressed() -> void:
for nodesData in get_tree().get_nodes_in_group("tableData"):
nodesData.call("rollStarted", len(diceScenes))
for nodesData in get_tree().get_nodes_in_group("tableActions"):
nodesData.call("startRoll")
for nodesData in get_tree().get_nodes_in_group("die"):
var tween: Tween = get_tree().create_tween()
tween.tween_property(nodesData.get_child(0), "scale", Vector3(0.01, 0.01, 0.01), 0.1)
await get_tree().create_timer(0.1).timeout
nodesData.free()
dieScene = preload("res://props/d6.tscn").instantiate()
add_child(dieScene)
dieScene.global_transform.origin = centerPoint.global_transform.origin
dieScene.global_transform.origin.z += 8
diceScenes.append(dieScene)
await update_dice_positions()
for die in diceScenes:
die.get_child(0).call("rollDie")
diceScenes = []
func update_dice_positions() -> void:
var count: int = len(diceScenes)
for index in range(count):
var die: Node = diceScenes[index]
var vec3: Vector3 = Vector3(centerPoint.global_transform.origin.x, centerPoint.global_transform.origin.y, centerPoint.global_transform.origin.z)
var tween: Tween = get_tree().create_tween()
tween.tween_property(die, "position", vec3, 0.08)
await get_tree().create_timer(0.08).timeout
There a bit more to unpack here.
What does this script do when _on_roll_pressed() is called? Simple:
- Tell everybody that the roll has started
- Clean every D6 still on the table
- Instantiate a new D6 and add it to our project tree
- Update its position
- Call rollDie function in the new D6
Step 1 will make sure our UI is not showing anything, or allowing the button to being pressed mid roll.
Step 2: We remove our D6 still active, and add a simple shrink animation using tweens before freeing it from our scene:
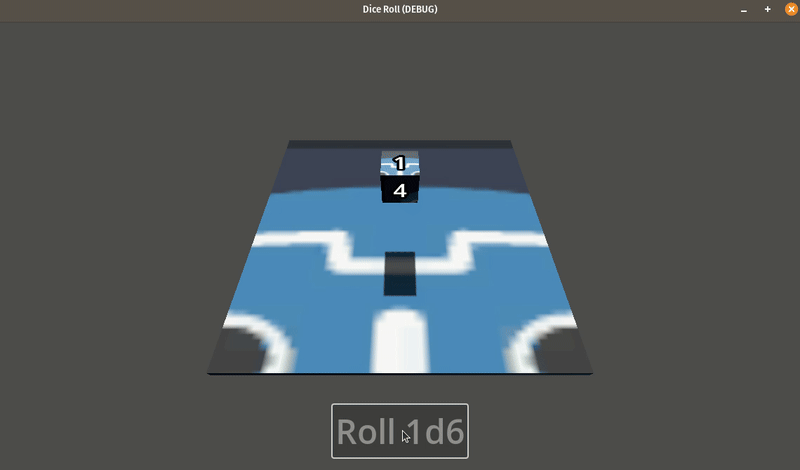
Step 3: Pretty straight forward, I load and instantiate the D6 resource, and add a new child. Here, we use the centerPoint variable, responsible to determine where the object will be instantiated.
Step 4: We call the update_dice_positions() here. This function also uses tweens, adding a floating animation for our new object:
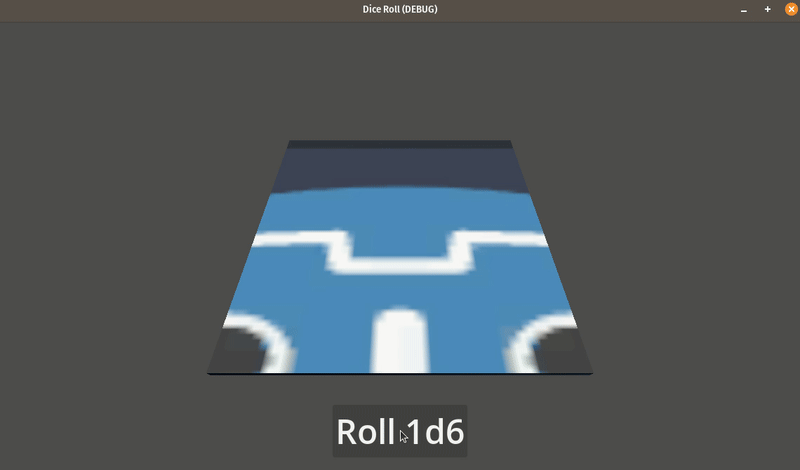
Step 5: For each new node stored on diceScenes, we call rollDie, realling starting the logic for a dice roll present in out D6 scene.
Note: The variable diceScenes exists because, during the scrapped project for the game jam, we could roll multiple dices:
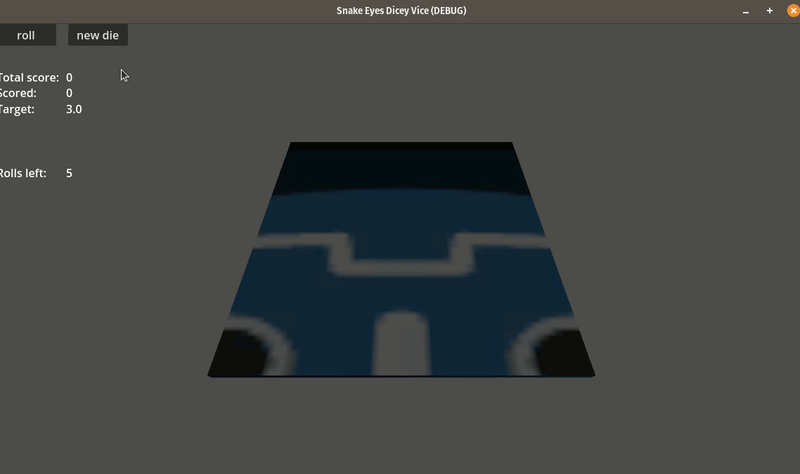
There are multiple ways you could implement this, but this will serve as a diary on how I approached this problem.
And about the implementation, all I will say is:
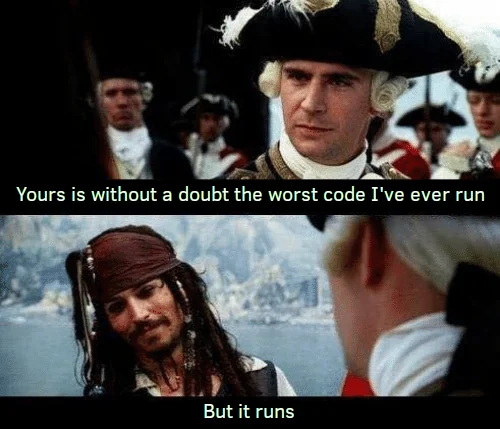